Learn how to test the KYC flow on iOS, covering setup, automated and manual testing, CI/CD integration, and handling common challenges effectively.

1. Introduction
Know Your Customer (KYC) is a crucial process for businesses, especially in the financial sector, to verify the identity of their clients. It involves collecting and verifying various forms of identification documents and information. This process helps prevent fraud, money laundering, and other illegal activities. In mobile apps, particularly those offering financial services, a streamlined and secure KYC process is essential.
The implementation of KYC processes is not limited to financial institutions. Non-financial apps, such as e-commerce platforms, digital wallets, and even social media applications, may require KYC to ensure the safety and security of their users. For example, a mobile wallet app needs to verify the identity of its users to prevent fraudulent transactions and comply with regulatory requirements. By understanding the nuances of the KYC process and how to effectively test it, you can ensure your app meets regulatory standards and provides a seamless user experience.
2. Setting Up the Testing Environment
Prerequisites for Testing KYC Flow on iOS
To effectively test the KYC flow on iOS, you need to ensure that your development and testing environments are properly set up. Here are the key prerequisites:
- Apple Developer Account: Ensure you have access to an Apple Developer account. This will allow you to deploy and test your application on physical iOS devices.
- Xcode: Install the latest version of Xcode. Xcode is Apple's integrated development environment (IDE) for macOS, used for developing software for iOS.
- iOS Devices: Having a variety of iOS devices is crucial. Different devices and iOS versions may exhibit different behaviors.
- Test Data: Prepare a set of test data that includes valid and invalid user information, documents, and scenarios to test the KYC flow comprehensively.
Tools and Frameworks Needed
Testing the KYC flow requires a combination of tools and frameworks to cover both automated and manual testing effectively:
- XCTest: This is the primary testing framework provided by Apple. It allows you to write unit tests, UI tests, and performance tests. XCTest is well-integrated with Xcode.
Example:
Swift
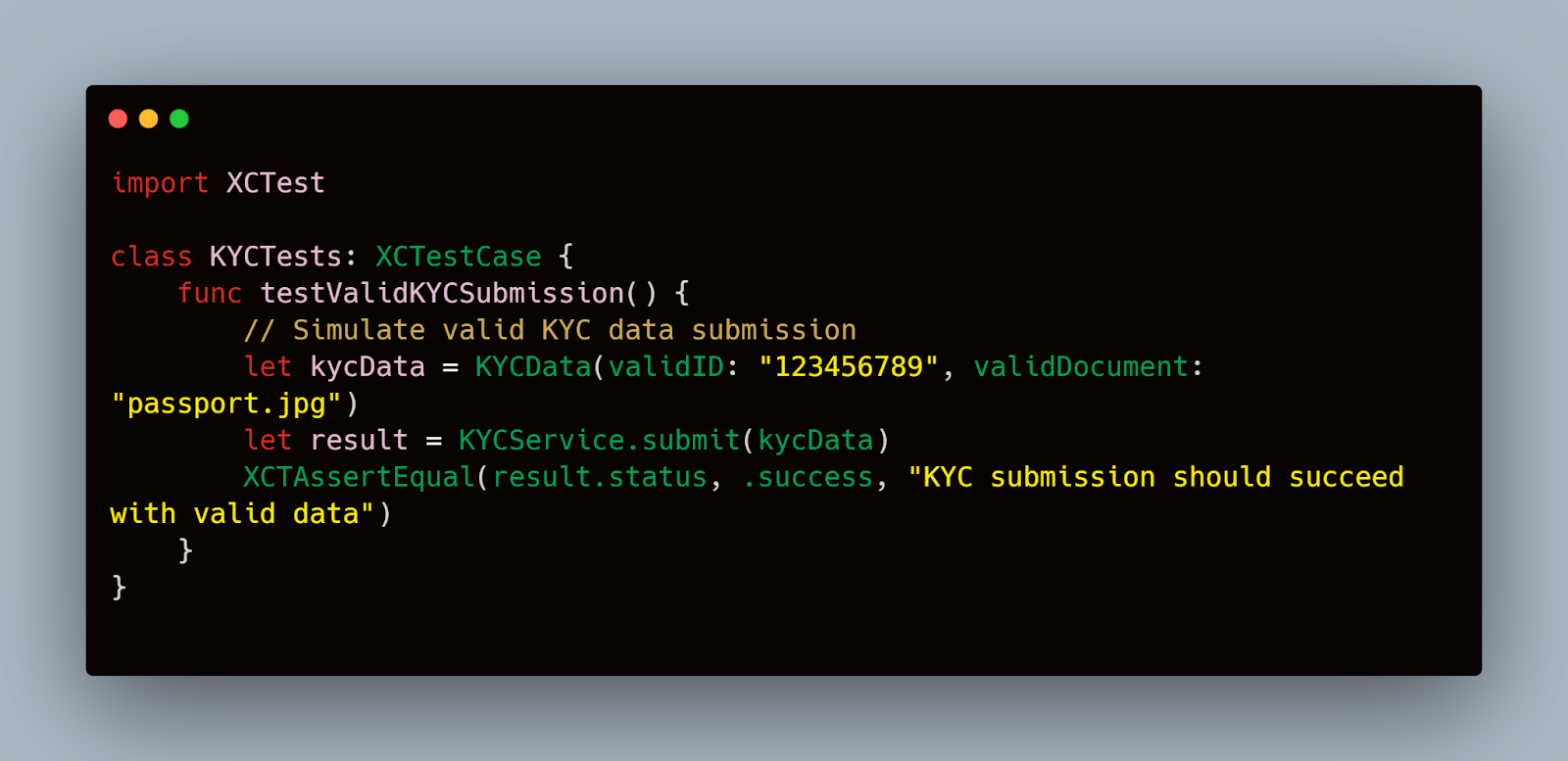
2. Appium: For cross-platform testing or if you prefer using a different testing tool, Appium is an excellent choice. It allows you to write tests in various programming languages and run them on multiple platforms, including iOS.
3. Mobot: Mobot's services can assist in automating repetitive and complex tasks in the KYC process. Mobot can simulate real-world interactions with your app, such as taking photos of documents and uploading them, which is crucial for testing the KYC flow.
4. Postman: Useful for testing the backend APIs involved in the KYC process. You can use Postman to simulate API calls and validate responses.
5. Charles Proxy: Helpful for monitoring and debugging network traffic between the app and the server. This tool is essential for ensuring that data transmitted during the KYC process is secure and correctly formatted.
Example Setup
- Install Xcode:
- Download and install Xcode from the Mac App Store.
- Ensure that you have the command-line tools installed by running xcode-select --install in the terminal.
- Set Up Devices:
- Connect your iOS devices to your Mac.
- In Xcode, go to Window > Devices and Simulators to add and manage your devices.
- Prepare Test Data:
- Create a folder with test documents (e.g., IDs, passports, utility bills).
- Ensure you have both valid and invalid documents for comprehensive testing.
- Configure XCTest:
- Create a new XCTest target in your Xcode project.
- Write test cases for each step in the KYC flow, such as document upload, facial recognition, and form submission.
- Example Test Case:
Swift

5. Integrate with Mobot:
- If using Mobot, ensure that your test scripts can interact with Mobot's APIs to automate tasks like document capture and submission.
By setting up your testing environment with these tools and frameworks, you will be well-equipped to test the KYC flow on iOS efficiently and effectively. This foundational setup will pave the way for thorough testing, ensuring your app's KYC process is reliable and user-friendly.
3. Understanding the KYC Flow
Typical Steps Involved in a KYC Process
The KYC process generally involves several key steps designed to verify the identity of a user. While the specifics can vary depending on the application and regulatory requirements, the typical KYC flow includes:
- User Registration: The process starts with the user providing basic information such as their name, email, and phone number.
- Identity Verification: The user is prompted to submit identification documents like a passport, driver's license, or national ID card.
- Address Verification: The user may be required to provide proof of address, such as a utility bill or bank statement.
- Biometric Verification: Some KYC processes include biometric verification, such as facial recognition or fingerprint scanning.
- Document Review: The submitted documents are reviewed by the system or manually by a compliance team.
- Approval/Rejection: Based on the review, the user is either approved or rejected for further use of the app's services.
User Scenarios and Edge Cases to Consider
When testing the KYC flow on iOS, it's essential to account for various user scenarios and edge cases to ensure comprehensive coverage. Here are some common scenarios and edge cases to consider:
- Valid User Information:
- Scenario: A user submits all required documents correctly, and the system approves the KYC.
- Test: Ensure that valid submissions lead to successful verification.
- Example Code:
swift

2. Invalid User Information:
- Scenario: A user submits incorrect or incomplete documents.
- Test: Ensure that the system correctly identifies and rejects invalid submissions.
- Example Code:
Swift
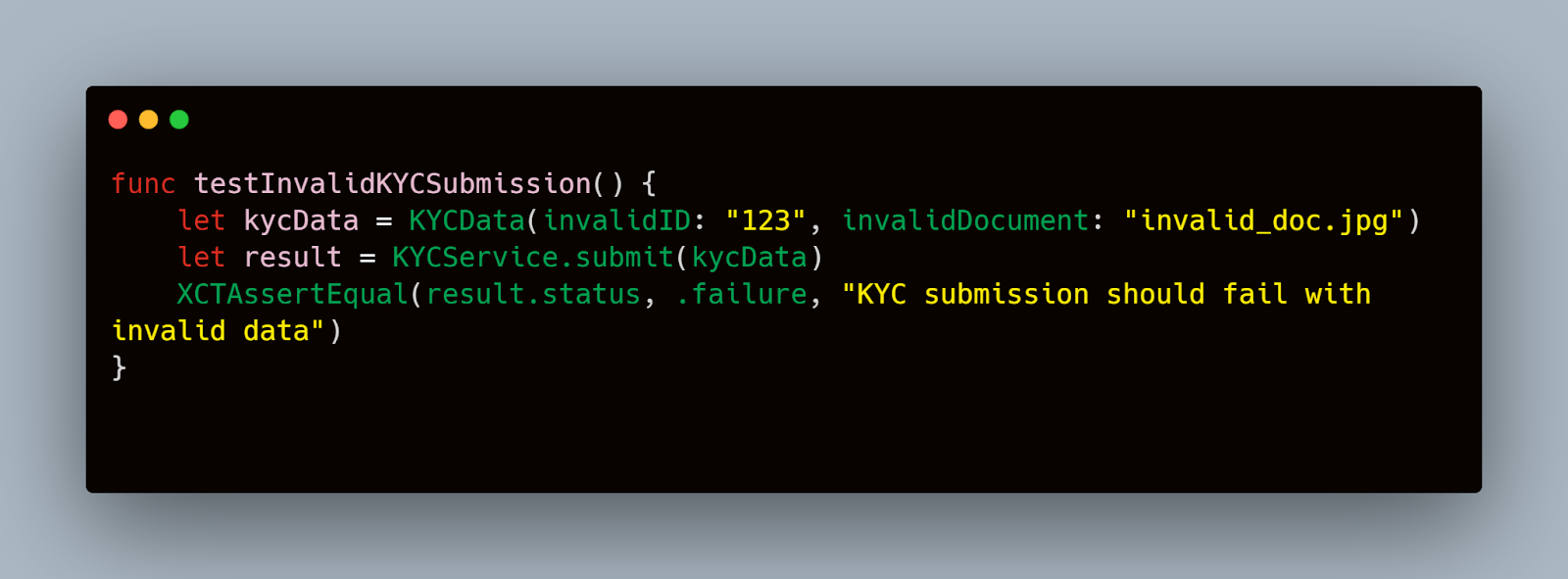
3. Network Issues:
- Scenario: The network connection drops during the document upload process.
- Test: Ensure that the app handles network interruptions gracefully and allows the user to retry.
- Example Code:
Swift

4. Biometric Verification Failure:
- Scenario: Biometric verification fails due to poor lighting or incorrect positioning.
- Test: Ensure the app provides clear instructions and retry options for biometric verification.
- Example Code:
Swift

5. Document Format Issues:
- Scenario: The user uploads a document in an unsupported format.
- Test: Ensure that the app detects unsupported formats and prompts the user to upload in the correct format.
- Example Code:

By covering these scenarios and edge cases, you can ensure that the KYC process in your iOS app is robust and user-friendly. This thorough testing approach helps in identifying and fixing potential issues before they impact the end users. Additionally, leveraging tools like Mobot can assist in automating repetitive tasks in these scenarios, providing more reliable and efficient test results.
4. Test Planning and Strategy
Identifying Test Cases for KYC Flow
Testing the KYC flow on iOS requires a detailed test plan that covers various scenarios and edge cases. Identifying the right test cases is crucial to ensure that all aspects of the KYC process are thoroughly tested. Here are some specific test cases to consider:
- Successful KYC Submission:
- User submits valid documents and completes the KYC process without any issues.
- Example Test Case:
swift
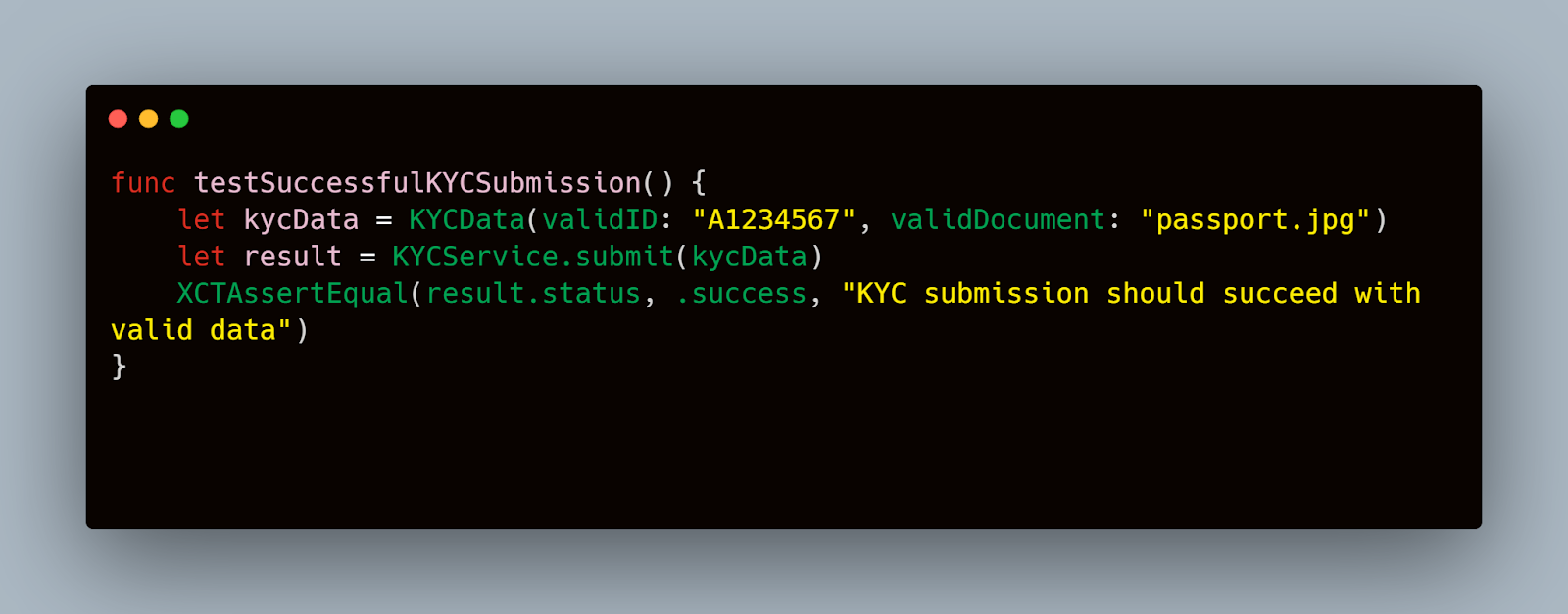
2. Incomplete Document Submission:
- User submits only part of the required documents (e.g., ID without proof of address).
- Example Test Case:
Swift

3. Invalid Document Format:
- User uploads documents in unsupported formats or with low resolution.
- Example Test Case:

4. Biometric Verification Failures:
- Biometric verification fails due to poor lighting or incorrect positioning of the face.
- Example Test Case:
Swift

5. Network Interruption:
- Network connectivity issues during document upload or verification.
- Example Test Case:
Swift
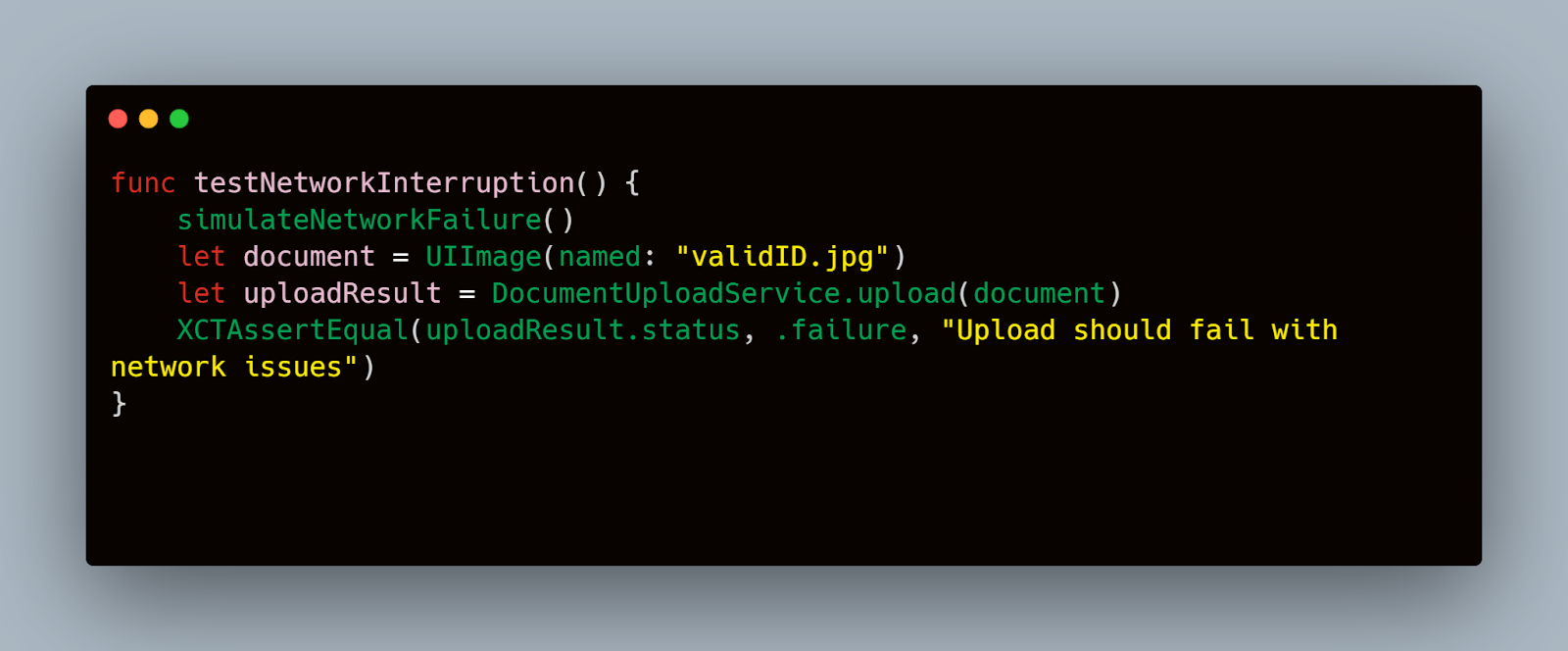
Defining the Scope of Manual vs. Automated Testing
Balancing manual and automated testing is crucial for an efficient and thorough testing strategy. Here’s how you can approach this:
- Automated Testing:
- Focus on repetitive tasks and scenarios that require frequent regression testing.
- Use XCTest for writing automated test cases that can be run regularly.
- Integrate with CI/CD pipelines to ensure tests run automatically on each build.
- Manual Testing:
- Reserve for complex scenarios and edge cases that require human judgment.
- Perform exploratory testing to uncover issues not covered by automated tests.
- Validate the user experience and ensure the app is intuitive and user-friendly.
While this guide emphasizes a hands-on approach to KYC testing, leveraging tools like Mobot can enhance your testing strategy. Mobot can automate parts of the KYC process, such as capturing and uploading documents, which can be repetitive and time-consuming. This allows QA engineers to focus on more critical testing aspects and ensures consistency in test execution.
Example Test Automation Strategy
- Setup Continuous Integration:
- Use a CI tool like Jenkins or Bitrise to automate the build and test process.
- Configure the CI tool to trigger tests on each code commit or pull request.
- Write and Maintain Test Scripts:
- Write XCTest scripts for common KYC scenarios.
- Regularly update and refactor test scripts to keep up with changes in the KYC process.
Example CI Configuration for Bitrise:
Yaml
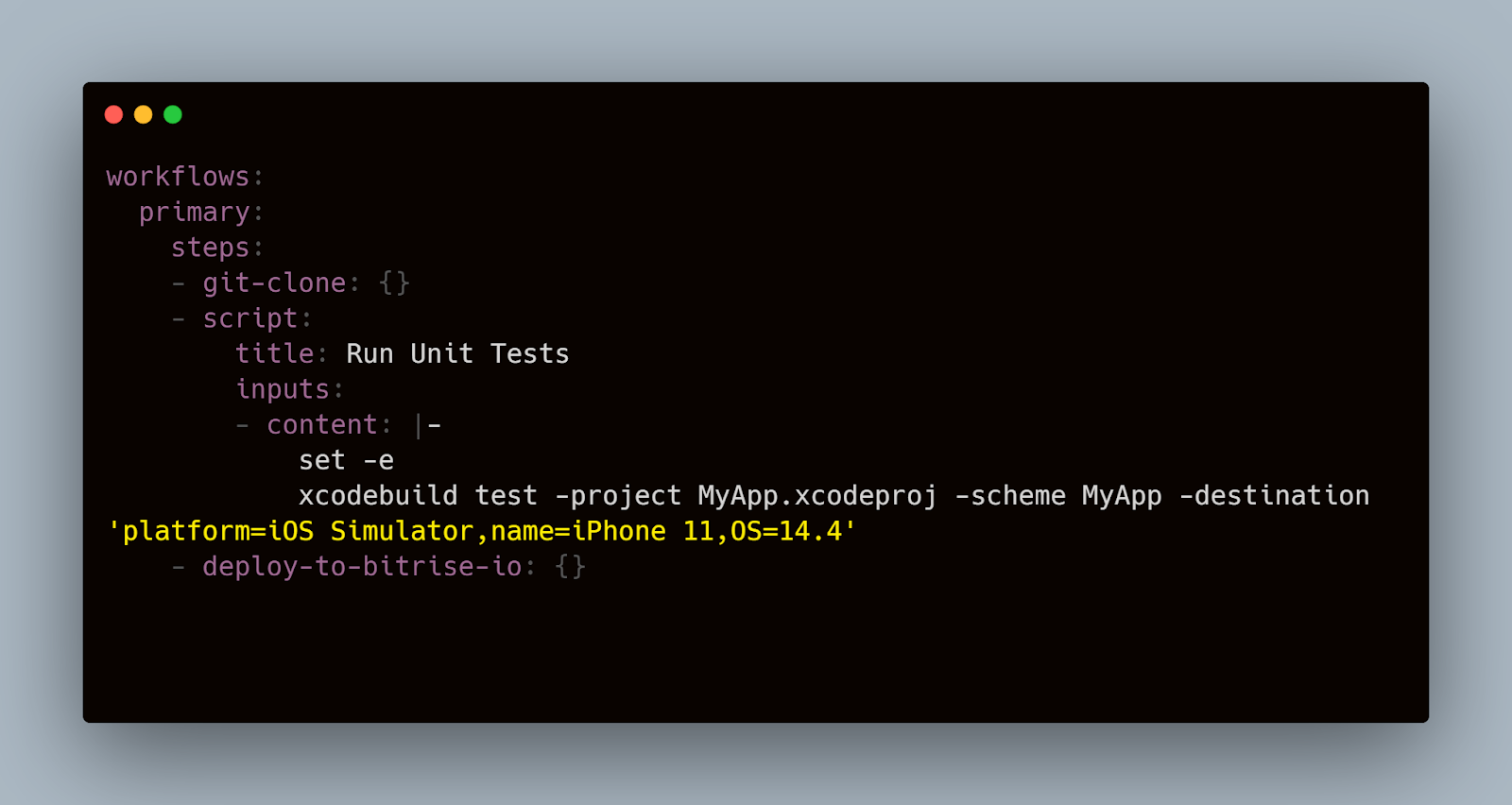
3. Monitor and Analyze Test Results:
- Use test reporting tools to monitor test results and identify flaky tests.
- Analyze test failures and prioritize fixes based on the impact on the KYC flow.
By carefully planning and strategizing your KYC flow testing, you can ensure comprehensive coverage and maintain a high level of quality in your iOS application. Leveraging a mix of manual and automated testing, along with tools like Mobot, will help streamline the testing process and improve overall efficiency.
5. Implementing Automated Tests
Automated tests are essential for ensuring the reliability and efficiency of the KYC process on iOS. By automating repetitive tasks, you can focus on more complex scenarios and maintain consistent test coverage. In this section, we will explore how to write automated tests using XCTest, a framework provided by Apple for testing iOS applications.
Writing Test Scripts Using XCTest
XCTest is the default testing framework for iOS applications. It allows you to write unit tests, UI tests, and performance tests. Here's how you can implement automated tests for different steps in the KYC flow.
- Setting Up XCTest in Your Project
First, ensure that your Xcode project is configured to include XCTest. Create a new test target if it doesn't already exist:
Swift
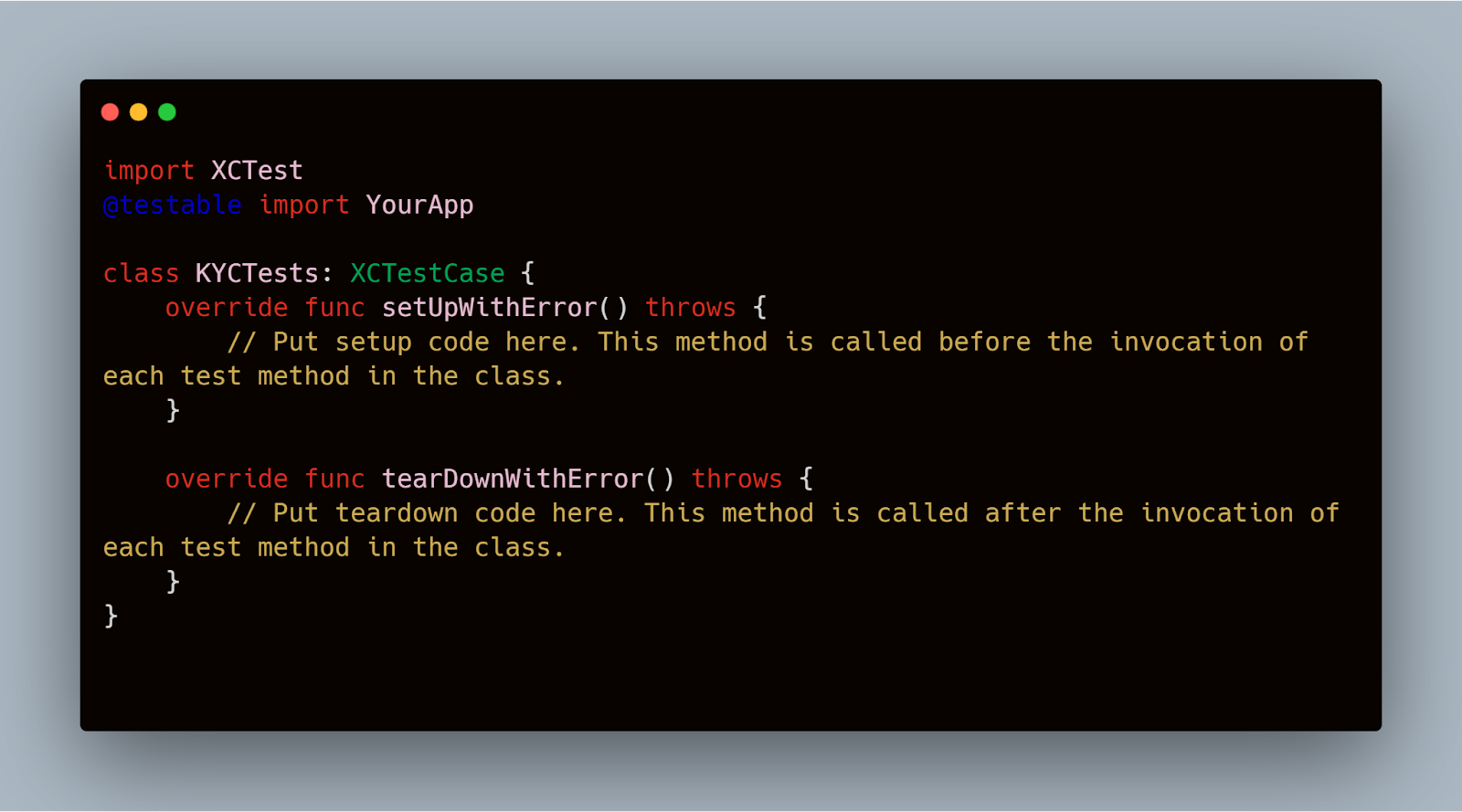
2. Writing Test Cases for KYC Steps
a. Document Upload Test
This test checks if the user can successfully upload a valid document.
Swift

b. Facial Recognition Test
This test ensures that the biometric verification process works correctly.
Swift
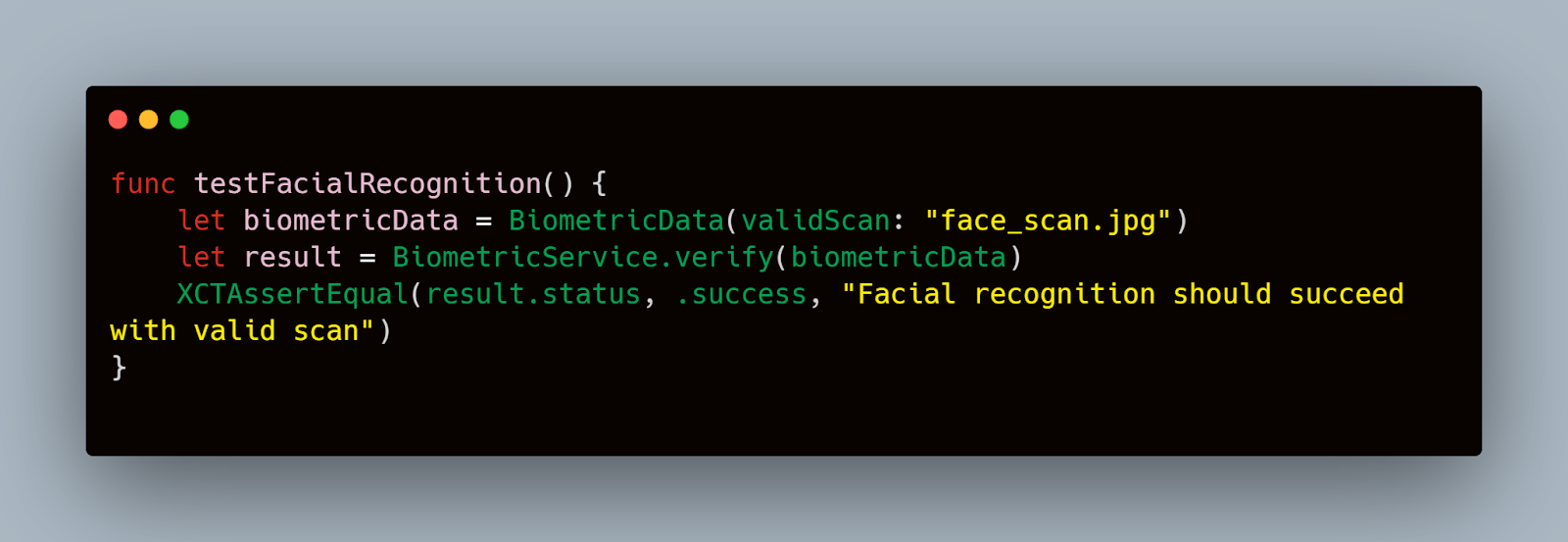
c. Form Submission Test
This test verifies that the user can submit the KYC form with all the required information.
Swift

3. Handling Errors and Edge Cases in Test Scripts
It's crucial to handle various errors and edge cases to ensure your tests are robust.
a. Network Failure Simulation
This test simulates a network failure during document upload and ensures the app handles it gracefully.
Swift
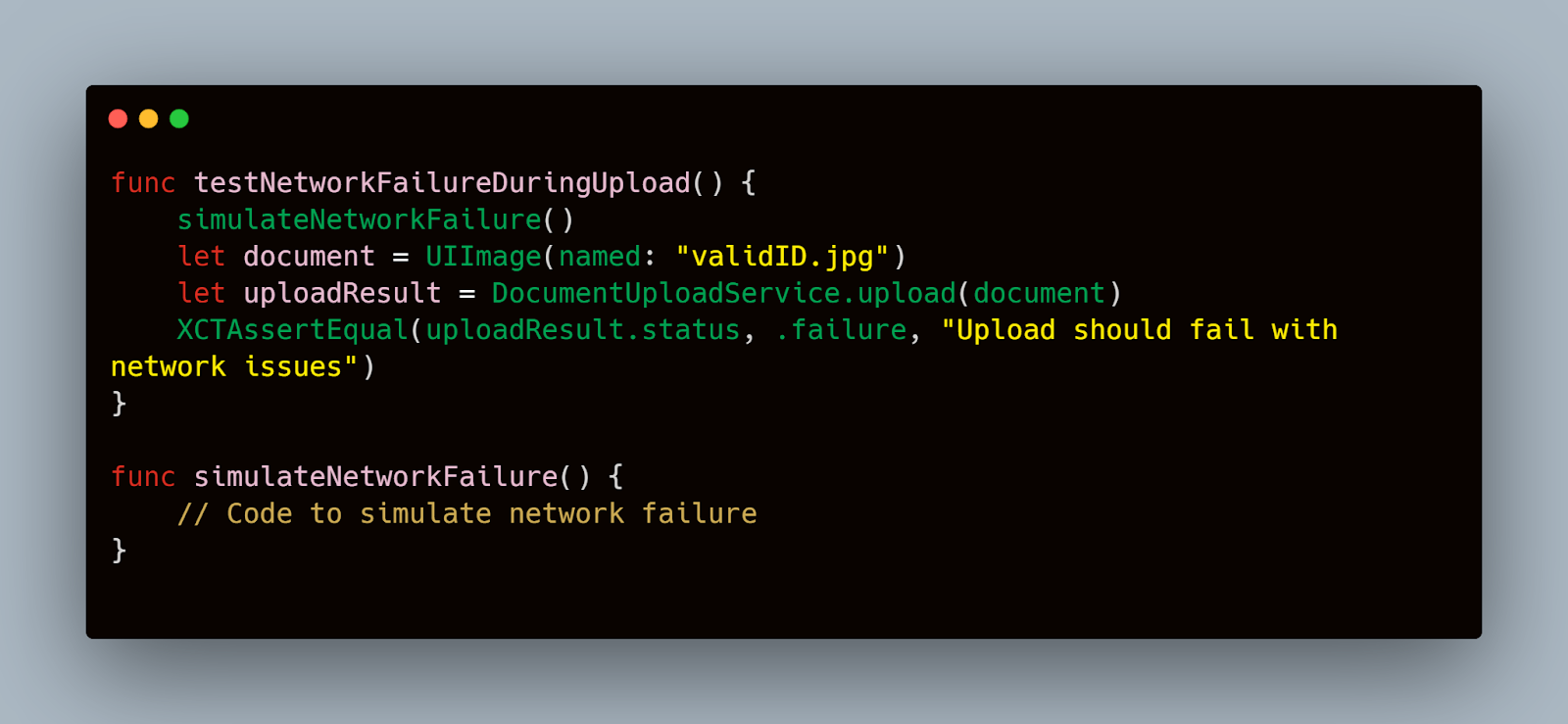
b. Invalid Document Format
This test checks if the app correctly handles the upload of unsupported document formats.
Swift
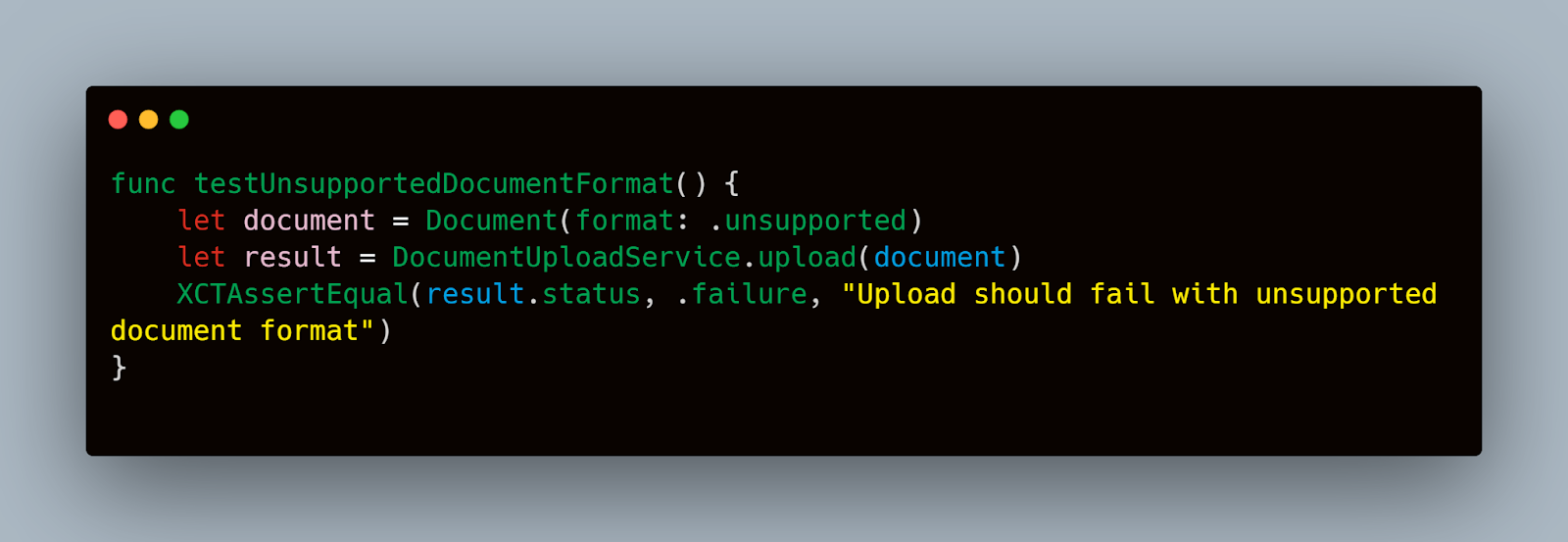
4. Integrating with CI/CD Pipelines
To ensure that your tests run automatically with every build, integrate them into your CI/CD pipeline. Here's an example configuration for Jenkins:
Jenkinsfile Example:
Groovy

By implementing these automated tests, you can ensure that the KYC flow in your iOS app is reliable and performs well under various conditions. Automated tests help catch issues early, reducing the risk of bugs reaching production and enhancing the overall user experience.
6. Manual Testing Best Practices
Manual testing is crucial for scenarios that require human judgment, exploratory testing, and covering edge cases that automated tests might miss. In this section, we will discuss best practices for performing manual testing on the KYC flow in iOS apps.
When and How to Perform Manual Testing
Manual testing should be strategically used to complement automated testing. Here are key scenarios where manual testing is essential:
- User Experience Testing:
- Validate that the KYC process is intuitive and user-friendly.
- Ensure that instructions are clear and that the user can easily navigate through the KYC steps.
- Example:
Markdown
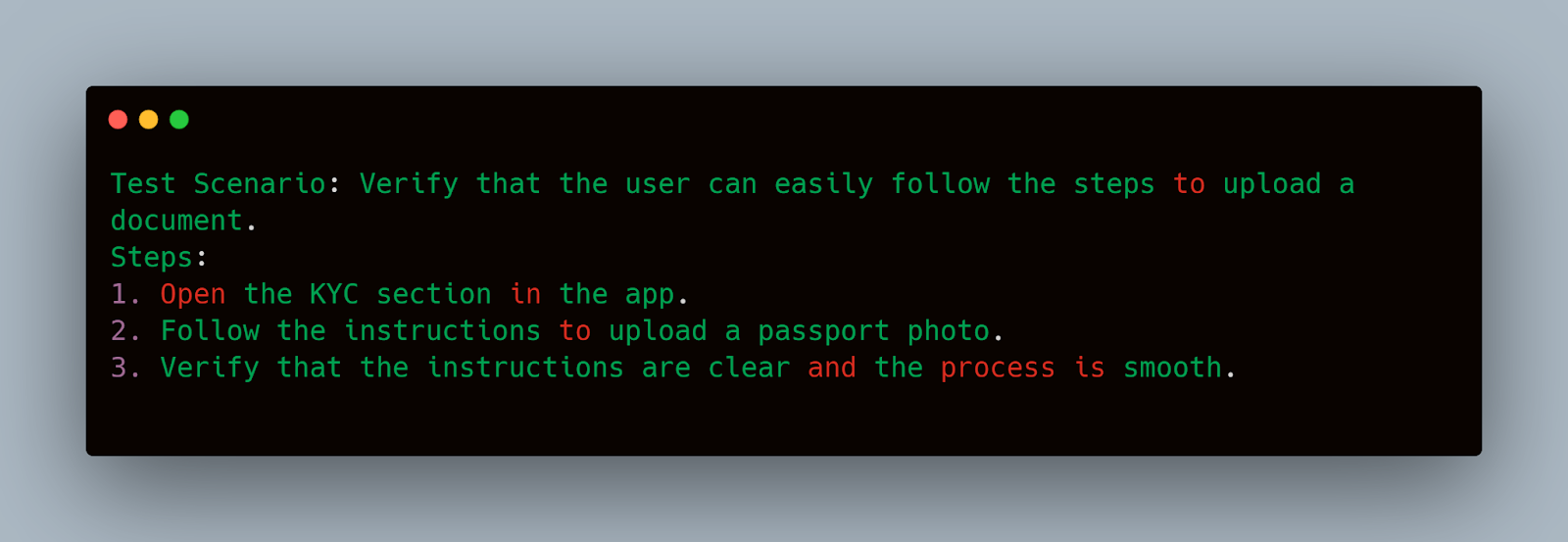
2. Exploratory Testing:
- Identify potential issues by testing beyond predefined scenarios.
- Perform ad-hoc testing to find bugs that automated tests might miss.
- Example:
Markdown
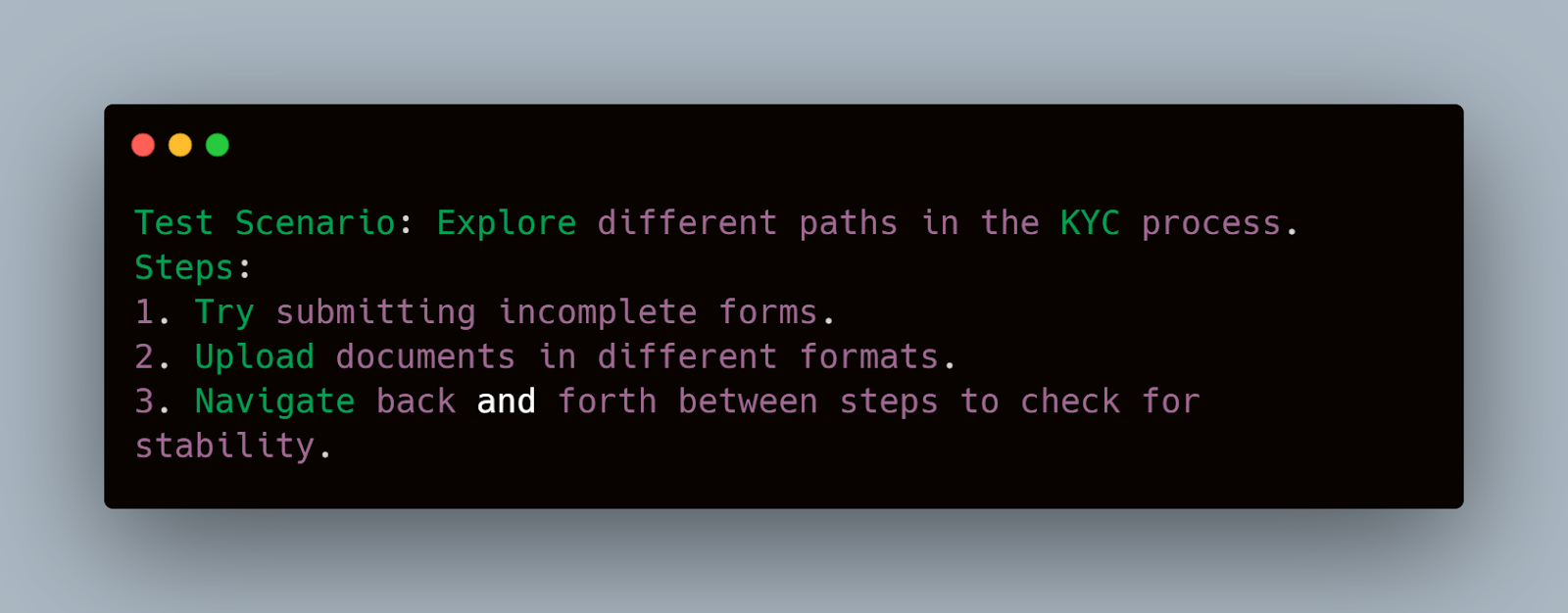
3. Complex Scenarios and Edge Cases:
- Test scenarios involving complex data or rare conditions.
- Example:
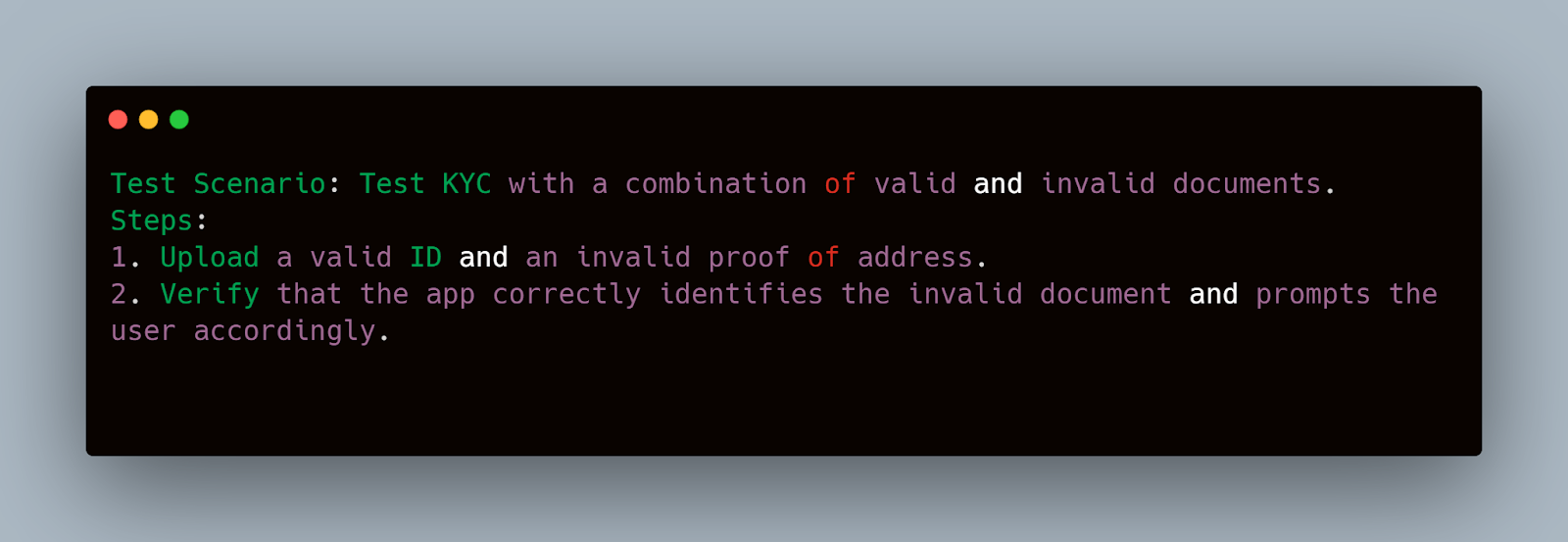
Ensuring Coverage of Edge Cases and Complex Scenarios
To ensure thorough testing of the KYC flow, it is important to cover various edge cases and complex scenarios:
- Multiple Document Types:
- Test the KYC process with different types of identification documents.
- Verify that the app supports various formats and types (e.g., passports, driver’s licenses, national IDs).
- Localized Testing:
- Test the KYC process with different languages and regional settings.
- Ensure that the app correctly handles localization and provides accurate translations.
- Accessibility Testing:
- Verify that the KYC process is accessible to users with disabilities.
- Ensure that the app supports screen readers, voice-over, and other accessibility features.
- Example Accessibility Test:
Markdown

Reporting and Documenting Findings
Accurate and detailed reporting is essential for addressing issues found during manual testing. Here are some best practices for documenting your findings:
- Use a Consistent Format:
- Document issues in a clear and structured format.
- Include steps to reproduce, expected results, actual results, and any relevant screenshots or logs.
- Example Issue Report:
Markdown

2. Use a Bug Tracking Tool:
- Utilize a tool like JIRA, Trello, or Asana to track and manage reported issues.
- Assign issues to the relevant team members and monitor their resolution status.
3. Communicate Findings Effectively:
- Share documented issues with the development team promptly.
- Provide clear and actionable feedback to facilitate quick resolution.
By following these manual testing best practices, you can ensure comprehensive coverage of the KYC flow, identify potential issues early, and improve the overall quality of your iOS application. Manual testing, combined with automated testing, provides a robust approach to verifying the KYC process, enhancing both security and user experience.
7. Continuous Integration and Testing
Integrating your KYC flow tests into a Continuous Integration (CI) and Continuous Deployment (CD) pipeline is crucial for maintaining the quality and reliability of your iOS application. This section will guide you through setting up and optimizing CI/CD pipelines to automate your KYC flow tests.
Integrating KYC Flow Tests into CI/CD Pipelines
- Choosing the Right CI/CD Tools
- Popular CI/CD tools for iOS development include Jenkins, Bitrise, and GitHub Actions.
- Choose a tool that integrates well with your development environment and supports iOS testing.
- Configuring Your CI/CD Pipeline
a. Jenkins Configuration Jenkins is a widely used open-source automation server that supports building, deploying, and automating iOS applications- Install Jenkins: Install Jenkins on a server or use a cloud-hosted Jenkins service.
- Install Required Plugins: Ensure you have the necessary plugins for iOS development, such as the Xcode plugin.
- Jenkinsfile Example:
Groovy

b. Bitrise Configuration Bitrise is a CI/CD platform designed specifically for mobile apps. It offers easy setup and a variety of integrations.
- Set Up a New App: Create a new app on Bitrise and connect your repository.
- Configure Workflows: Define workflows for building, testing, and deploying your app.
Bitrise Workflow Example:
Yaml
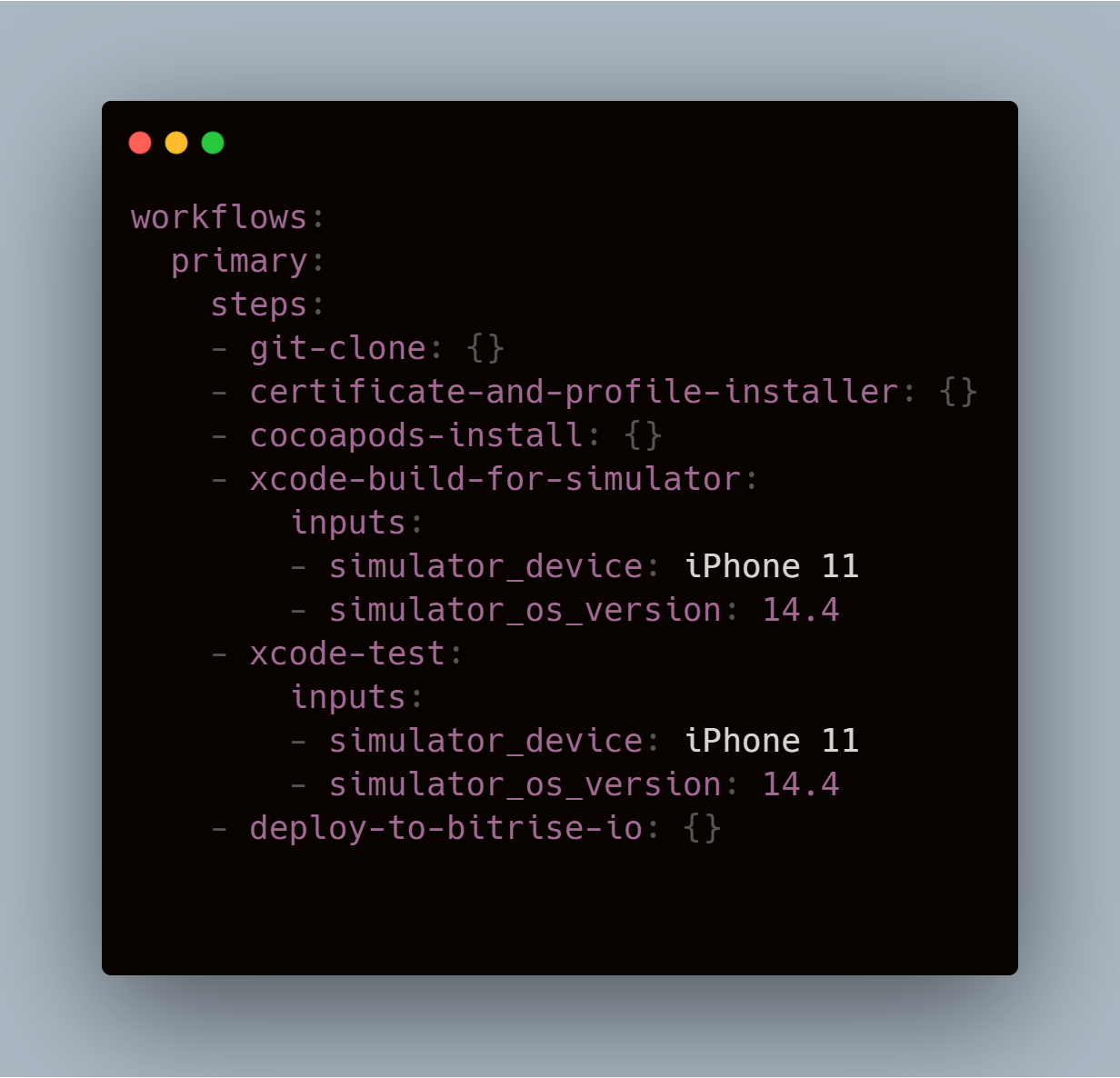
3. Running Tests Automatically
- Trigger Builds on Code Changes: Configure your CI/CD pipeline to trigger builds and tests automatically whenever code is pushed to the repository or a pull request is created.
- Parallel Test Execution: Utilize parallel test execution to speed up the testing process, especially if you have a large suite of tests.
4. Analyzing Test Results
- JUnit Reports: Use JUnit reports to visualize test results within your CI/CD dashboard. This helps in quickly identifying failed tests and addressing issues.
- Email Notifications: Set up email notifications or Slack integrations to alert your team of test failures or build issues.
Example JUnit Configuration in Jenkins:
Groovy
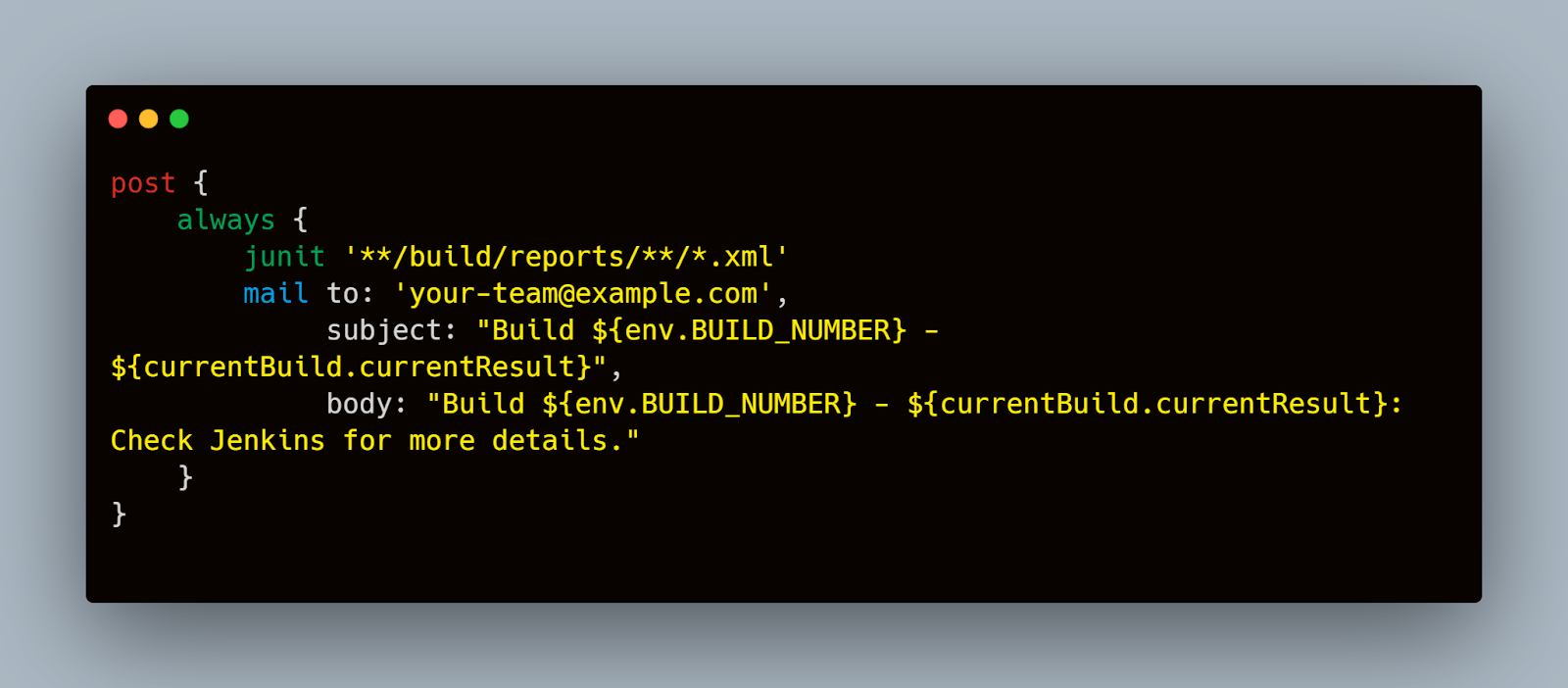
5. Best Practices for CI/CD Testing
- Keep Your Test Suite Fast: Ensure your tests are optimized for speed to maintain quick feedback loops.
- Maintain Test Stability: Regularly update and refactor your test scripts to adapt to changes in the application and reduce flaky tests.
- Security Considerations: Secure your CI/CD pipelines to prevent unauthorized access and ensure sensitive data is handled securely.
By integrating KYC flow tests into your CI/CD pipeline, you ensure that your application is continuously tested, maintaining high quality and reliability. Automated tests running as part of your CI/CD process catch issues early, reducing the risk of bugs reaching production and enhancing user trust in your application.
8. Common Challenges and Solutions
Testing the KYC flow on iOS can present several challenges, from dealing with network issues to handling flaky tests. In this section, we will explore common challenges you might encounter and practical solutions to address them effectively.
Handling Network Issues and API Errors
Network stability and API reliability are critical factors in the KYC process. Here’s how to handle these challenges:
- Simulating Network Conditions:
- Use tools like Network Link Conditioner to simulate various network conditions (e.g., slow connections, interruptions) during testing.
- Example:
Swift

2. Handling API Errors:
- Ensure your application gracefully handles API errors by providing clear error messages and allowing retries.
- Example:

3. Retry Logic:
- Implement and test retry logic for API calls to handle transient network issues.
- Example:
Swift

Dealing with Flaky Tests and Intermittent Failures
Flaky tests can undermine the reliability of your test suite. Here are strategies to minimize flaky tests:
- Stabilizing UI Tests:
- Ensure that UI tests wait for elements to appear before interacting with them.
- Use XCTest expectations to wait for asynchronous operations to complete.
- Example:
Swift
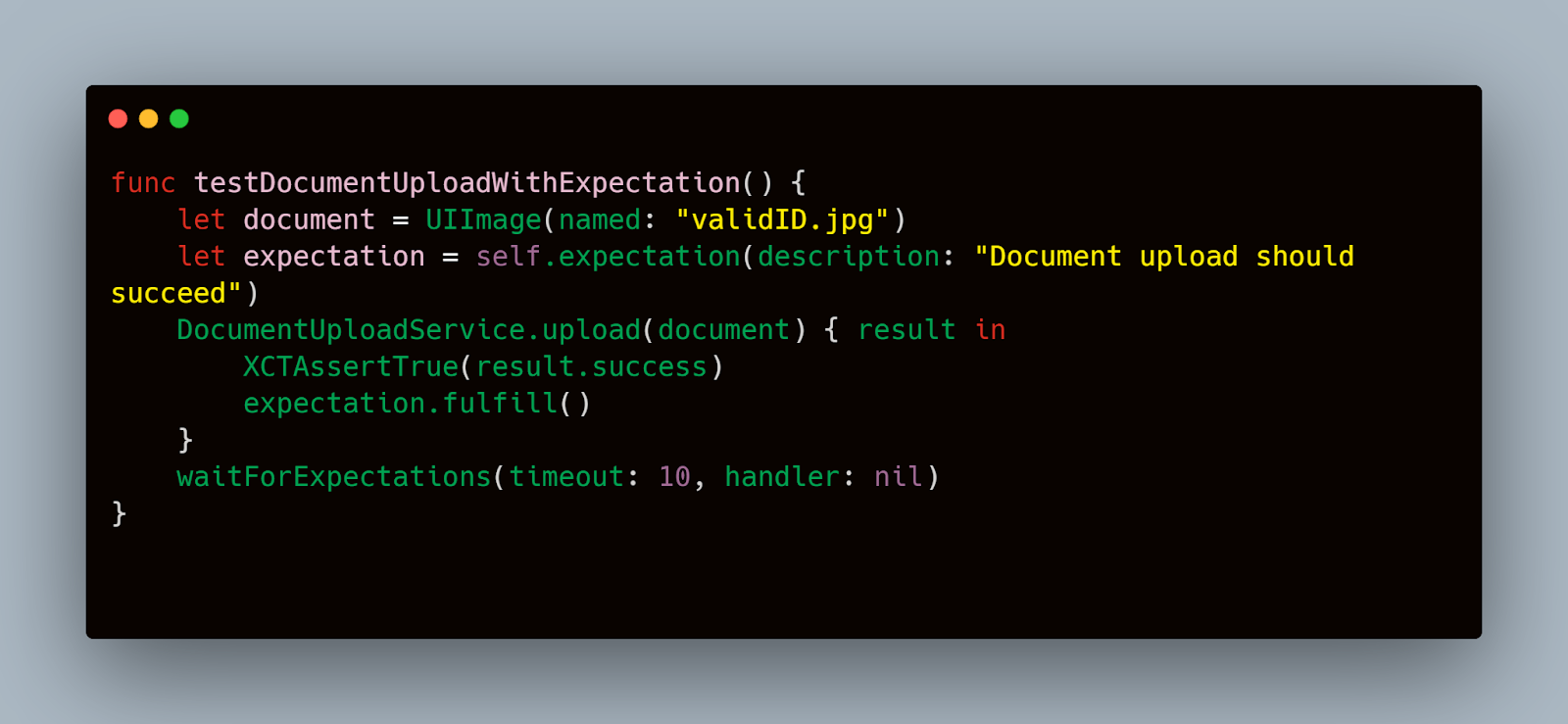
2. Isolating Tests:
- Make sure tests are independent and do not rely on shared state.
- Clean up any side effects after each test to maintain test isolation.
- Example:
Swift
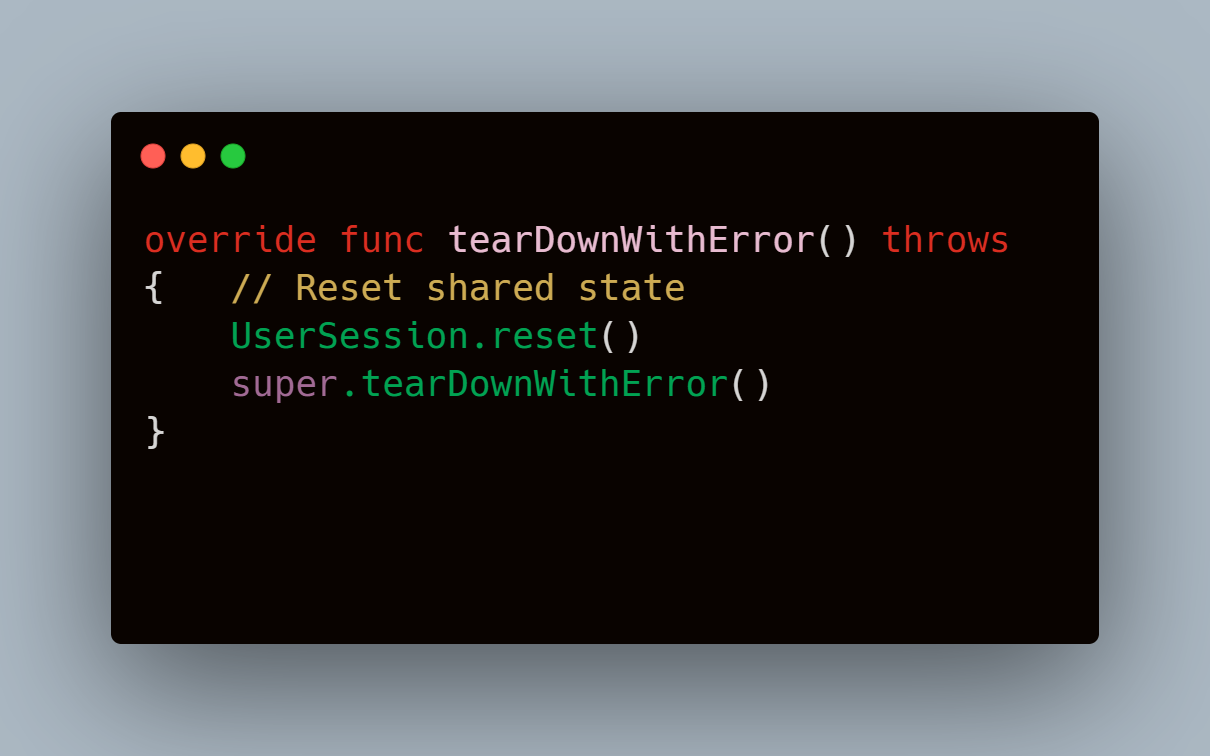
3. Mocking External Dependencies:
- Use mocks and stubs to isolate tests from external dependencies such as network services.
- Example:
Swift

Best Practices for Maintaining Test Stability
Maintaining stable tests over time requires ongoing effort and best practices:
- Regular Test Review:
- Periodically review and refactor tests to ensure they remain relevant and effective.
- Remove or update tests that no longer provide value due to changes in the application.
- Continuous Monitoring:
- Monitor test results to identify patterns in failures.
- Use dashboards and alerts to stay informed about test stability issues.
- Collaboration and Feedback:
- Foster a culture of collaboration between QA and development teams.
- Encourage developers to write and maintain tests for their code.
- Provide feedback on test results and work together to address flaky tests and failures.
By addressing these common challenges with practical solutions, you can enhance the reliability and effectiveness of your KYC flow tests on iOS. This comprehensive approach ensures that your application provides a secure and seamless KYC experience for users.
9. Conclusion
Ensuring a secure and efficient KYC process in your iOS application is crucial for compliance and user trust. By implementing comprehensive test strategies that combine both manual and automated testing, you can cover all critical aspects of the KYC flow. Leveraging tools like XCTest for automation, addressing common challenges, and integrating tests into your CI/CD pipeline ensures that your application maintains high quality and reliability. Remember, a well-tested KYC flow not only enhances security but also improves the overall user experience, contributing to the success and credibility of your application.