Learn how to effectively test OAuth sign-in on iOS with practical strategies, tools, and advanced techniques to enhance security and usability.

IntroductionÂ
OAuth is a widely adopted authorization framework that enables applications to obtain limited access to user accounts on an HTTP service, such as Facebook, GitHub, or Google. Its implementation allows for secure delegated access, meaning that it lets applications act on behalf of a user without accessing their passwords.
For iOS developers, integrating OAuth can streamline the user experience by simplifying how users sign in and access data while ensuring security and privacy. However, the process is not without its challenges, particularly when it comes to testing. Ensuring that the OAuth sign-in process works smoothly and securely on iOS devices requires a thorough understanding of both the OAuth protocol and the iOS operating environment.
OAuth's significance in iOS development stems from its ability to protect user credentials and provide a token-based authentication system. This system allows users to grant applications access to the information stored by third-party services without exposing their credentials. For instance, an app might use OAuth to access a user's data from social media platforms without requiring them to share their username and password.
Common Challenges with OAuth on iOS
Implementing OAuth on iOS devices involves several common challenges:
- Token Management: Safely storing and managing refresh tokens for long-term sessions can be complex.
- Security: Ensuring that the OAuth implementation is secure against common vulnerabilities, such as insecure redirect URIs and cross-site request forgery (CSRF) attacks.
- User Experience: Maintaining a smooth flow that handles various authentication states, such as login, logout, and token renewal, without degrading the user experience.
Setting Up the Environment
Before getting into How to Test OAuth Sign-In on iOS, it’s important to set up the right environment. To effectively test OAuth sign-in mechanisms on iOS, it's essential to establish a proper testing environment that replicates real-world conditions as closely as possible. This setup involves several components, including the necessary tools, configuration settings, and test accounts. By preparing your environment meticulously, you can ensure that your tests are both efficient and comprehensive.
Tools and Accounts Required
- Xcode: Apple’s integrated development environment (IDE) is crucial for building and testing iOS applications. Ensure you have the latest version installed to take advantage of the newest features and security patches.
- Simulator and Physical Devices: Testing should occur on both the iOS Simulator (part of Xcode) and actual iOS devices to understand how your application behaves in different hardware configurations.
- OAuth Provider Setup: You'll need access to the OAuth provider that your application uses. This could be a social media platform like Facebook or a Google account, depending on your application’s requirements. Ensure you have valid credentials and the necessary permissions to conduct tests.
- Network Monitoring Tools: Tools like Charles Proxy or Wireshark are invaluable for monitoring and debugging the network calls made by your application during the OAuth process.
Configuring Your iOS Application for OAuth Testing
- Setting Up the Redirect URI: The redirect URI is a critical component of the OAuth flow. It must be configured both in your iOS application and on the OAuth provider’s dashboard to ensure that tokens are correctly redirected back to your application after authentication.
- Client ID and Secret: Register your application with the OAuth provider to obtain a client ID and secret. These must be securely stored and used to authenticate requests from your application to the OAuth provider.
- Configuring Info.plist: Your iOS project’s Info.plist file needs to include any URL schemes and external URLs involved in the OAuth process. This setup is crucial for handling redirects properly.
- Implementing OAuth Libraries: Consider using established libraries like OAuthSwift or AlamofireOAuth2 to handle OAuth integrations. These libraries provide a strong framework for managing authentication flows, token storage, and error handling.
Example Configuration
Here's a basic setup using OAuthSwift, a popular library for implementing OAuth in Swift applications:
swift
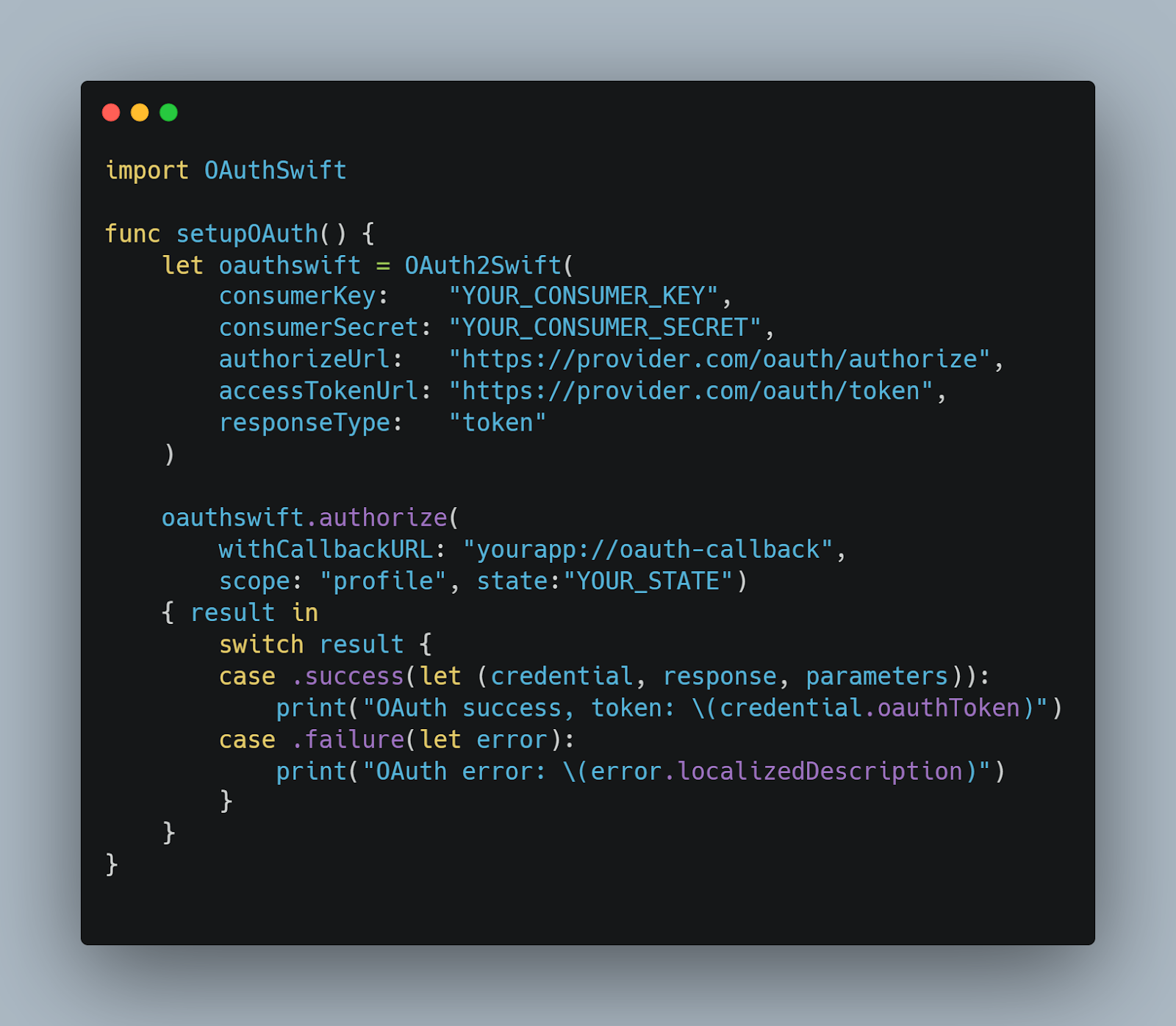
This snippet initializes an OAuth2Swift object with the necessary credentials and URLs, and starts the authorization process with a callback URL that handles the redirect after successful authentication.
By setting up your environment as described, you prepare your iOS application for thorough testing of its OAuth functionalities. This preparation helps in identifying potential issues early in the development cycle, ultimately leading to a more reliable and secure application.
Leveraging Mobot for OAuth Testing
While manual and automated testing play crucial roles in the development cycle, incorporating an advanced testing service like Mobot can further enhance the strength of OAuth implementations. Mobot specializes in simulating realistic user interactions, which are critical for testing OAuth sign-in processes that involve complex user flows and multiple redirections. This section explains how integrating Mobot into your testing strategy can improve test coverage and reliability.
Enhancing Realistic User Simulations
Mobot uses physical robots to interact with mobile devices, providing a layer of testing that mimics real user behaviors more accurately than software simulations. This approach is particularly beneficial for OAuth testing, where real-world interactions, such as entering credentials and handling pop-up windows, are pivotal.
Example Scenario Using Mobot:
- Multi-Factor Authentication Flow: Testing OAuth sign-in that includes multi-factor authentication (MFA) can be tricky, especially when it involves biometrics or hardware tokens. Mobot can be programmed to perform these actions, such as tapping a security key or scanning a fingerprint, to ensure the process works seamlessly.
Consider a case where an iOS app uses OAuth for integration with multiple social media platforms. Each platform might have slightly different authentication flows or require different user interactions.
- Setup: Configure Mobot to handle multiple devices and platforms. Program it to navigate through the OAuth sign-in process for each platform.
- Execution: Mobot performs the sign-in process, mimicking a real user by typing in usernames and passwords, handling redirects, and interacting with any multi-factor authentication that might be required.
- Validation: Mobot provides detailed logs and video recordings of each test session, allowing developers to see exactly how the app behaved during the tests. This data is crucial for identifying UI or flow issues that might not be evident in automated software tests.
Integrating Mobot into Your Development Pipeline
Incorporating Mobot into your continuous integration/continuous delivery (CI/CD) pipeline can significantly streamline the testing process. Here’s how to integrate Mobot:
- Test Planning: Define which parts of the OAuth flow are critical and could benefit from realistic user interaction testing.
- Configuration: Set up Mobot with your iOS application, specifying the exact steps and interactions required for each test.
- Execution: Run tests using Mobot as part of your regular build process or on a scheduled basis.
- Review: Analyze the results from Mobot’s testing. Focus on areas where user interaction issues occur, and adjust your application’s OAuth flow accordingly.
By leveraging Mobot’s capabilities, teams can not only reduce the time and effort required to test complex OAuth scenarios but also significantly increase confidence in their application’s functionality across various real-world conditions. This method provides a comprehensive understanding of how the application performs under actual user conditions, which is often challenging to achieve with traditional testing methodologies alone.
OAuth Testing Strategies
Testing OAuth implementations in iOS apps is critical to ensure security and functionality.Â
Unit tests are essential for verifying the discrete components of your OAuth integration. Focus on testing the authentication logic, token handling, and error management separately from the network.
- Token Generation and Expiry Handling: Write tests to verify that tokens are generated correctly and that token expiry is handled gracefully. For instance, your app should request a new token seamlessly when the current one expires.
- Error Handling: Simulate various failure scenarios, such as invalid credentials or network failures, to ensure your app handles these gracefully. Check that appropriate error messages are displayed and that the app does not crash.
Here's an example of a unit test in Swift using XCTest that checks token refresh logic:
swift

Integration Testing with the OAuth Provider
Integration tests ensure that your application interacts correctly with the OAuth provider. These tests should simulate real-world usage as closely as possible.
- Complete Authentication Flow: Test the entire authentication process from start to finish. Ensure that after the user logs in, the correct data is retrieved using the OAuth tokens.
- Redirect Handling: Verify that your app correctly handles redirects from the OAuth provider, capturing the tokens passed via URL parameters or fragments.
Example of an integration test scenario:
swift

Scenario-Based Testing
Beyond functional testing, scenario-based tests help you understand how your application behaves under specific conditions:
- Multiple Account Handling: Test scenarios where users might switch between different OAuth providers or accounts.
- Consent Withdrawal: Simulate the user withdrawing consent from the OAuth provider's side and verify that your app handles such situations by requesting re-authentication.
- Network Conditions: Test how your app handles various network conditions, including slow or intermittent connectivity, to ensure that OAuth functionalities are strong under all circumstances.            Â
By employing these testing strategies, you ensure that your iOS application's OAuth sign-in process is not only compliant with standards but also provides a secure, efficient user experience. Each test case contributes to building a reliable application that maintains user trust and meets business requirements.
Automating OAuth Tests
Automation is a key strategy in modern software testing, offering both efficiency and thoroughness, particularly for repetitive tasks like OAuth sign-in testing.
Tools and Frameworks for Automation
Several tools can facilitate the automation of OAuth tests in iOS applications. Here’s a brief overview:
- XCTest Framework: Apple's native XCTest framework supports both unit and UI tests. It can be integrated seamlessly into your CI/CD pipeline, ensuring that OAuth functionalities are tested automatically with each build.
- Appium: A cross-platform test automation tool that can handle OAuth tests involving native, mobile web, and hybrid applications. Appium scripts interact with iOS apps just as a user would, by simulating taps, swipes, and other user actions.
- Postman: While primarily used for API testing, Postman can automate the testing of OAuth token generation and refresh mechanisms by making direct HTTP requests to the OAuth server.
Setting Up Automated Tests
Automating an OAuth test involves simulating the user's interaction with the OAuth provider. Here is a basic outline using XCTest:
Swift
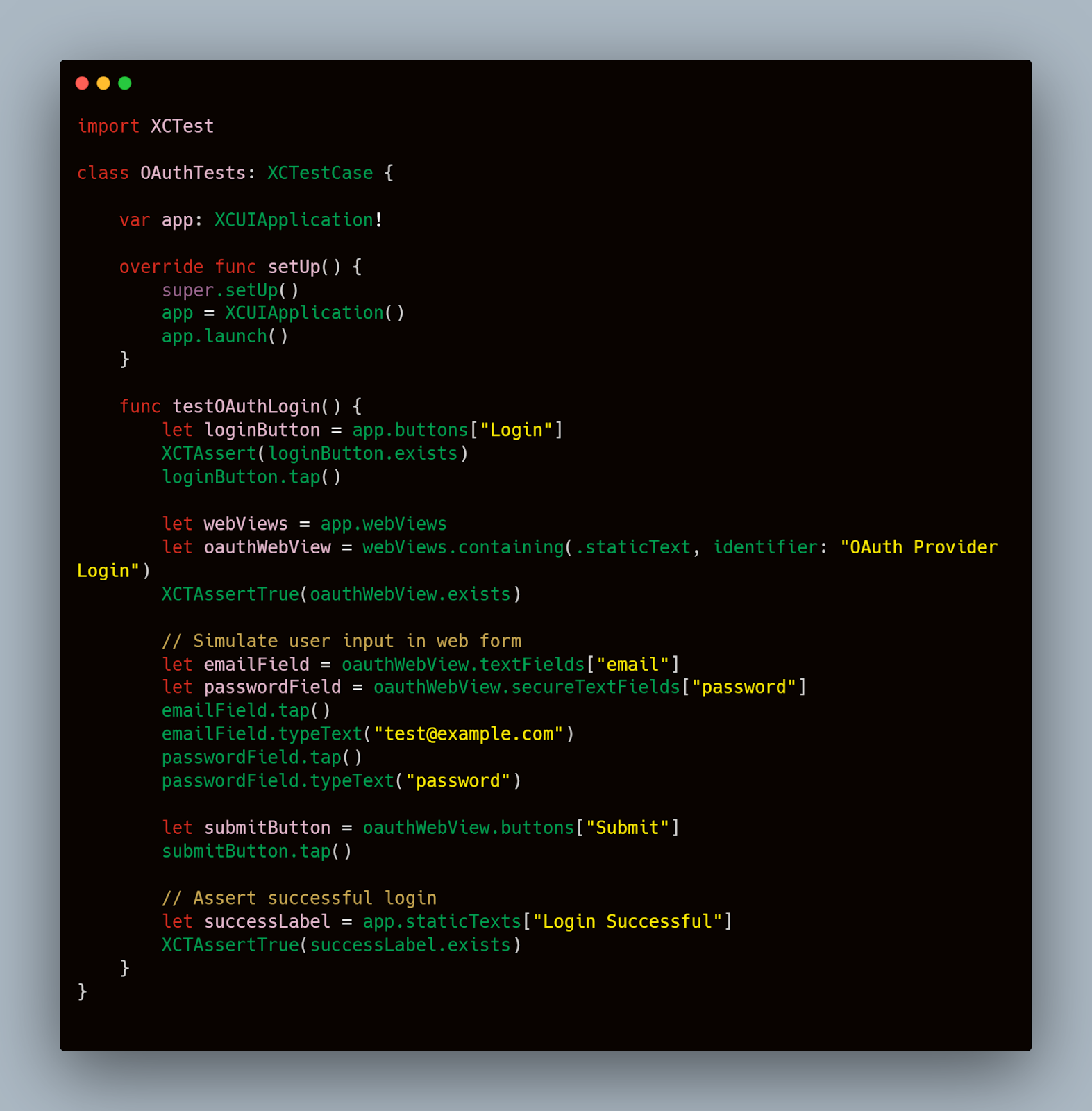
This test script simulates a user tapping the login button, entering their credentials into the OAuth provider's web form, and submitting the form. It then checks for a label indicating a successful login, confirming that the OAuth flow works as expected.
Advantages of Automating OAuth Tests
Automating your OAuth tests offers several benefits:
- Consistency: Tests perform the same operations in the same order every time they are run, reducing human error.
- Repeatability: Tests can be run as often as needed – after every build, nightly, or right before a release.
- Speed: Automation significantly speeds up the process of testing OAuth implementations, especially across different device configurations and operating systems.
By incorporating these automation strategies into your testing routine, you can ensure that your application's OAuth integration is strong, secure, and delivers a seamless user experience. Automation not only saves time but also provides a level of assurance that manual testing alone cannot achieve, making it an indispensable part of the OAuth testing process in iOS development.
Common OAuth Issues and How to Debug Them
When implementing OAuth in iOS applications, developers often encounter specific issues that can hinder the authentication process. Identifying and resolving these issues promptly ensures a smoother user experience and enhances security. This section covers common OAuth issues and provides practical tips for debugging them.
Troubleshooting Common OAuth Errors
- Invalid Token Errors: These errors occur when the access token is not accepted by the OAuth provider. It could be due to the token being expired, revoked, or malformed.
Debugging Steps:- Check the validity of the token in your application logs.
- Ensure that the system clock is synchronized, as a mismatch can often cause tokens to be seen as expired.
- Verify that the tokens are stored and transmitted securely without being altered.
- Redirection Issues: Problems with redirect URIs can prevent OAuth from completing successfully. This might manifest as the application not receiving the control back after authentication.
Debugging Steps:- Confirm that the redirect URI registered with the OAuth provider matches the one in your application exactly.
- Use network monitoring tools to trace the HTTP redirect flow and identify where it fails.
- User Consent Errors: Sometimes, users might not grant consent properly due to UI/UX issues or misunderstandings of the consent process.
Debugging Steps:- Review the user interface for obtaining consent to ensure it is clear and functioning correctly.
- Check logs to confirm that the consent step is triggering and completing as expected.
Using Logging and Network Monitoring Tools
Effective debugging often requires detailed logs and the ability to monitor network traffic. Tools like Charles Proxy or Wireshark can capture OAuth interactions, helping identify issues with request headers, payloads, and responses.
Example Setup Using Charles Proxy:
- Configure Charles Proxy to intercept HTTPS traffic from your iOS device.
- Start the OAuth process and monitor the traffic that flows to and from the OAuth provider.
- Look for discrepancies in request headers or unexpected status codes in responses.
Code Snippet for Logging OAuth Processes
Implementing comprehensive logging can help trace issues during OAuth transactions. Below is a Swift code snippet that sets up enhanced logging for OAuth-related operations:
Swift
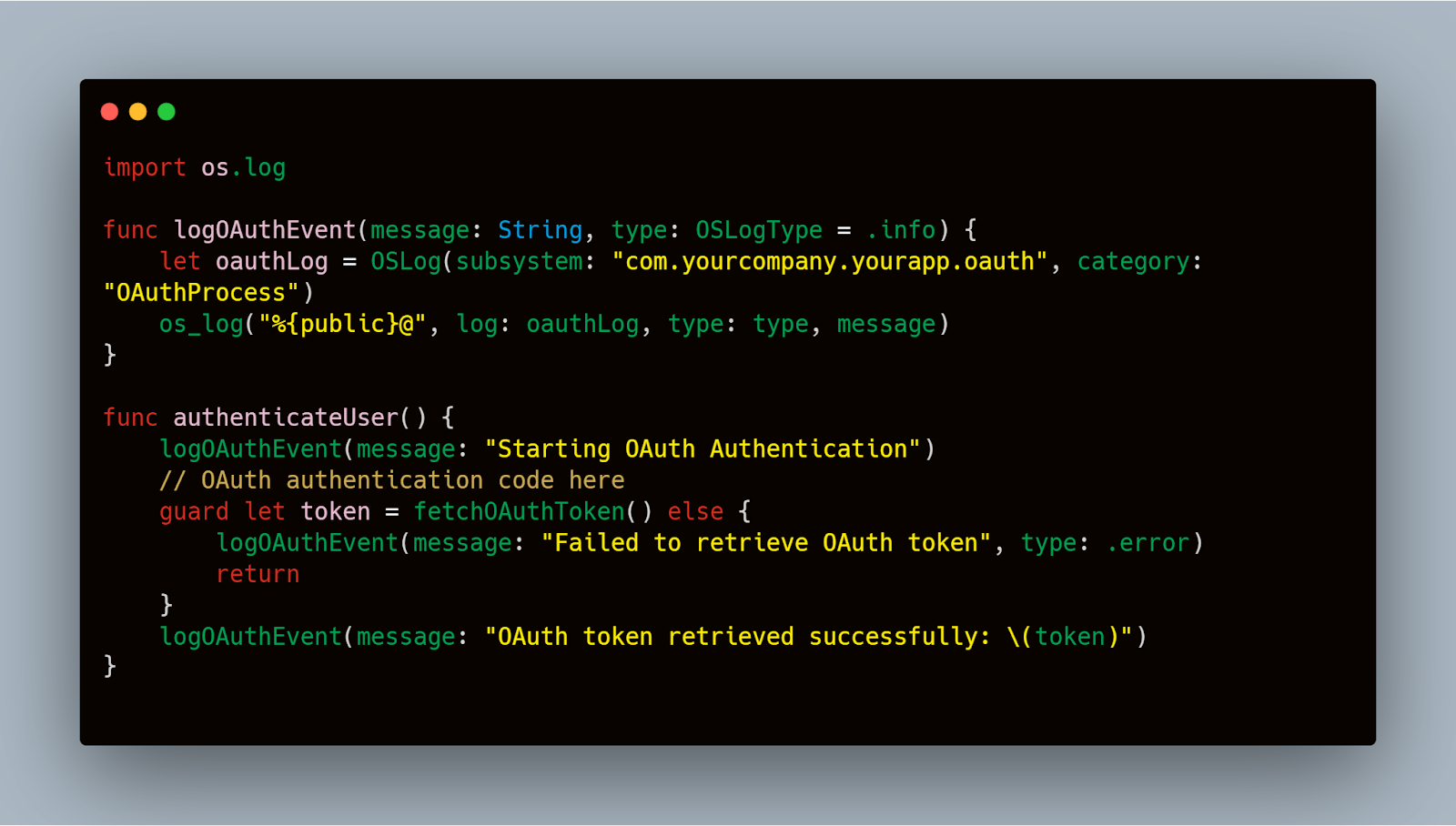
This snippet utilizes the os.log framework to log different events within the OAuth process, allowing for different log levels (info, error) to categorize the nature of the logged information effectively.
By employing these debugging strategies and tools, developers can quickly identify and rectify issues within their OAuth implementation, minimizing downtime and improving the security and usability of their iOS applications. These practices are crucial for maintaining strong OAuth systems that protect user data and enhance overall application performance.
Security Considerations
Incorporating OAuth into iOS applications entails not only enabling access through third-party services but also ensuring that such integrations adhere to stringent security standards. This section discusses key security practices for OAuth implementations and outlines how to test for vulnerabilities.
Security Best Practices for OAuth
- Secure Storage of Tokens: Tokens should be stored securely using iOS's Keychain Services, which provides a strong mechanism for encrypting and securing sensitive information on devices.
- Use of HTTPS: Ensure all communications with OAuth providers and your application servers are transmitted over HTTPS to protect data from interception during transit.
- Validation of Redirect URIs: Strictly validate redirect URIs to prevent malicious redirections that could lead to token interception or unauthorized access.
Testing for Common Security Vulnerabilities
The testing phase should focus on identifying and mitigating potential security flaws that could be exploited. Here are some common security checks:
- Testing for Leakage of Sensitive Information: Monitor and ensure that sensitive information, such as tokens and user credentials, is not logged or leaked in any manner that could be accessed by unauthorized parties.
- Injection Flaws: Test input fields that interact with the OAuth process for injection flaws. Ensure that the application securely handles input without exposing itself to cross-site scripting (XSS) or SQL injection attacks.
- Cross-Site Request Forgery (CSRF): Ensure that the application is protected against CSRF attacks, where an attacker could trick a user into executing unwanted actions on a web application in which they're authenticated.
Implementing Code Snippets for Security Tests
Here’s a code snippet using Swift and XCTest to test for token storage security:
Swift

This test ensures that the token is securely stored in and retrieved from the iOS Keychain, verifying that the token does not get exposed in less secure storage locations.
By rigorously applying these security best practices and testing methodologies, developers can significantly mitigate risks associated with OAuth implementations, ensuring that their iOS applications remain secure and trustworthy.
ConclusionÂ
Testing OAuth sign-in on iOS requires a comprehensive approach that includes both manual and automated strategies to ensure a secure and user-friendly authentication experience. By implementing the practices outlined in this article—from setting up your testing environment and employing rigorous testing strategies to leveraging advanced tools like Mobot for realistic user interaction simulations—you can significantly enhance the reliability and security of your OAuth implementations.Â
It's crucial to regularly update and refine your testing processes to adapt to new iOS updates and changes in OAuth standards. This continuous improvement will help maintain the strength of your authentication mechanisms, ultimately leading to a trustworthy and efficient application that meets the high standards expected by today's mobile users.
‍